Qt No Such Slot In Derived Class
This is the sequel of my previous article explaining the implementation details of the signals and slots.In the Part 1, we have seenthe general principle and how it works with the old syntax.In this blog post, we will see the implementation details behind thenew function pointerbased syntax in Qt5.
Like any other function, it defines who has access to call an instance's slot. Public ones can obviously be called by code outside of the class, whereas protected and private slots are accessible only within a class. And even then private slots are only available to that class, not any derived classes. @mrjj QObject::metaObject returns the QMetaObject associated with the class (it's a static member, and the method provides virtualization over the class tree). If you don't have the QOBJECT macro, there's no staticMetaObject member for the class, and consequently there's no metaObject override, thus you get the parent's staticMetaObject (or the last class that had the macro). One goal of this project is to explore the possibilities of creating generic code for use in Qt, such as the generic context menu class. There is no such thing in QML as generic concepts, everything that needs to be exposed to QtQuick needs to go through Qt land first, meaning I have to expose it either as its own QObject derived type.
New Syntax in Qt5
The new syntax looks like this:
Why the new syntax?
I already explained the advantages of the new syntax in adedicated blog entry.To summarize, the new syntax allows compile-time checking of the signals and slots. It also allowsautomatic conversion of the arguments if they do not have the same types.As a bonus, it enables the support for lambda expressions.
New overloads
There was only a few changes required to make that possible.
The main idea is to have new overloads to QObject::connect
which take the pointersto functions as arguments instead of char*
There are three new static overloads of QObject::connect
: (not actual code)
The first one is the one that is much closer to the old syntax: you connect a signal from the senderto a slot in a receiver object.The two other overloads are connecting a signal to a static function or a functor object withouta receiver.
They are very similar and we will only analyze the first one in this article.
Pointer to Member Functions
Before continuing my explanation, I would like to open a parenthesis totalk a bit about pointers to member functions.
Here is a simple sample code that declares a pointer to member function and calls it.
Pointers to member and pointers to member functions are usually part of the subset of C++ that is not much used and thus lesser known.
The good news is that you still do not really need to know much about them to use Qt and its new syntax. All you need to remember is to put the &
before the name of the signal in your connect call. But you will not need to cope with the ::*
, .*
or ->*
cryptic operators.
These cryptic operators allow you to declare a pointer to a member or access it.The type of such pointers includes the return type, the class which owns the member, the types of each argumentand the const-ness of the function.
You cannot really convert pointer to member functions to anything and in particular not tovoid*
because they have a different sizeof
.
If the function varies slightly in signature, you cannot convert from one to the other.For example, even converting from void (MyClass::*)(int) const
tovoid (MyClass::*)(int)
is not allowed.(You could do it with reinterpret_cast; but that would be an undefined behaviour if you callthem, according to the standard)
Pointer to member functions are not just like normal function pointers.A normal function pointer is just a normal pointer the address where thecode of that function lies.But pointer to member function need to store more information:member functions can be virtual and there is also an offset to apply to thehidden this
in case of multiple inheritance.sizeof
of a pointer to a member function can evenvary depending of the class.This is why we need to take special care when manipulating them.
Type Traits: QtPrivate::FunctionPointer
Let me introduce you to the QtPrivate::FunctionPointer
type trait.
A trait is basically a helper class that gives meta data about a given type.Another example of trait in Qt isQTypeInfo.
What we will need to know in order to implement the new syntax is information about a function pointer.
The template<typename T> struct FunctionPointer
will give us informationabout T via its member.
ArgumentCount
: An integer representing the number of arguments of the function.Object
: Exists only for pointer to member function. It is a typedef to the class of which the function is a member.Arguments
: Represents the list of argument. It is a typedef to a meta-programming list.call(T &function, QObject *receiver, void **args)
: A static function that will call the function, applying the given parameters.
Qt still supports C++98 compiler which means we unfortunately cannot require support for variadic templates.Therefore we had to specialize our trait function for each number of arguments.We have four kinds of specializationd: normal function pointer, pointer to member function,pointer to const member function and functors.For each kind, we need to specialize for each number of arguments. We support up to six arguments.We also made a specialization using variadic templateso we support arbitrary number of arguments if the compiler supports variadic templates.
The implementation of FunctionPointer
lies inqobjectdefs_impl.h.
QObject::connect
The implementation relies on a lot of template code. I am not going to explain all of it.
Here is the code of the first new overload fromqobject.h:
You notice in the function signature that sender
and receiver
are not just QObject*
as the documentation points out. They are pointers totypename FunctionPointer::Object
instead.This uses SFINAEto make this overload only enabled for pointers to member functionsbecause the Object
only exists in FunctionPointer
ifthe type is a pointer to member function.
We then start with a bunch ofQ_STATIC_ASSERT
.They should generate sensible compilation error messages when the user made a mistake.If the user did something wrong, it is important that he/she sees an error hereand not in the soup of template code in the _impl.h
files.We want to hide the underlying implementation from the user who should not needto care about it.
That means that if you ever you see a confusing error in the implementation details,it should be considered as a bug that should be reported.
We then allocate a QSlotObject
that is going to be passed to connectImpl()
.The QSlotObject
is a wrapper around the slot that will help calling it. It alsoknows the type of the signal arguments so it can do the proper type conversion.
We use List_Left
to only pass the same number as argument as the slot, which allows connectinga signal with many arguments to a slot with less arguments.
QObject::connectImpl
is the private internal functionthat will perform the connection.It is similar to the original syntax, the difference is that instead of storing amethod index in the QObjectPrivate::Connection
structure,we store a pointer to the QSlotObjectBase
.
The reason why we pass &slot
as a void**
is only tobe able to compare it if the type is Qt::UniqueConnection
.
We also pass the &signal
as a void**
.It is a pointer to the member function pointer. (Yes, a pointer to the pointer)
Signal Index
We need to make a relationship between the signal pointer and the signal index.
We use MOC for that. Yes, that means this new syntaxis still using the MOC and that there are no plans to get rid of it :-).
MOC will generate code in qt_static_metacall
that compares the parameter and returns the right index.connectImpl
will call the qt_static_metacall
function with thepointer to the function pointer.
Once we have the signal index, we can proceed like in the other syntax.
The QSlotObjectBase
QSlotObjectBase
is the object passed to connectImpl
that represents the slot.
Before showing the real code, this is what QObject::QSlotObjectBasewas in Qt5 alpha:
It is basically an interface that is meant to be re-implemented bytemplate classes implementing the call and comparison of thefunction pointers.
It is re-implemented by one of the QSlotObject
, QStaticSlotObject
orQFunctorSlotObject
template class.
Fake Virtual Table
The problem with that is that each instantiation of those object would need to create a virtual table which contains not only pointer to virtual functionsbut also lot of information we do not need such asRTTI.That would result in lot of superfluous data and relocation in the binaries.
In order to avoid that, QSlotObjectBase
was changed not to be a C++ polymorphic class.Virtual functions are emulated by hand.
The m_impl
is a (normal) function pointer which performsthe three operations that were previously virtual functions. The 're-implementations'set it to their own implementation in the constructor.
Please do not go in your code and replace all your virtual functions by such ahack because you read here it was good.This is only done in this case because almost every call to connect
would generate a new different type (since the QSlotObject has template parameterswich depend on signature of the signal and the slot).
Protected, Public, or Private Signals.
Signals were protected
in Qt4 and before. It was a design choice as signals should be emittedby the object when its change its state. They should not be emitted fromoutside the object and calling a signal on another object is almost always a bad idea.
However, with the new syntax, you need to be able take the addressof the signal from the point you make the connection.The compiler would only let you do that if you have access to that signal.Writing &Counter::valueChanged
would generate a compiler errorif the signal was not public.
In Qt 5 we had to change signals from protected
to public
.This is unfortunate since this mean anyone can emit the signals.We found no way around it. We tried a trick with the emit keyword. We tried returning a special value.But nothing worked.I believe that the advantages of the new syntax overcome the problem that signals are now public.
Sometimes it is even desirable to have the signal private. This is the case for example inQAbstractItemModel
, where otherwise, developers tend to emit signalfrom the derived class which is not what the API wants.There used to be a pre-processor trick that made signals privatebut it broke the new connection syntax.
A new hack has been introduced.QPrivateSignal
is a dummy (empty) struct declared private in the Q_OBJECTmacro. It can be used as the last parameter of the signal. Because it is private, only the objecthas the right to construct it for calling the signal.MOC will ignore the QPrivateSignal last argument while generating signature information.See qabstractitemmodel.h for an example.
More Template Code
The rest of the code is inqobjectdefs_impl.h andqobject_impl.h.It is mostly standard dull template code.
I will not go into much more details in this article,but I will just go over few items that are worth mentioning.
Meta-Programming List
As pointed out earlier, FunctionPointer::Arguments
is a listof the arguments. The code needs to operate on that list:iterate over each element, take only a part of it or select a given item.
That is why there isQtPrivate::List
that can represent a list of types. Some helpers to operate on it areQtPrivate::List_Select
andQtPrivate::List_Left
, which give the N-th element in the list and a sub-list containingthe N first elements.
The implementation of List
is different for compilers that support variadic templates and compilers that do not.
With variadic templates, it is atemplate<typename... T> struct List;
. The list of arguments is just encapsulatedin the template parameters.
For example: the type of a list containing the arguments (int, QString, QObject*)
would simply be:
Without variadic template, it is a LISP-style list: template<typename Head, typename Tail > struct List;
where Tail
can be either another List
or void
for the end of the list.
The same example as before would be:
ApplyReturnValue Trick
In the function FunctionPointer::call
, the args[0]
is meant to receive the return value of the slot.If the signal returns a value, it is a pointer to an object of the return type ofthe signal, else, it is 0.If the slot returns a value, we need to copy it in arg[0]
. If it returns void
, we do nothing.
The problem is that it is not syntaxically correct to use thereturn value of a function that returns void
.Should I have duplicated the already huge amount of code duplication: once for the voidreturn type and the other for the non-void?No, thanks to the comma operator.
In C++ you can do something like that:
You could have replaced the comma by a semicolon and everything would have been fine.
Where it becomes interesting is when you call it with something that is not void
:
There, the comma will actually call an operator that you even can overload.It is what we do inqobjectdefs_impl.h
ApplyReturnValue is just a wrapper around a void*
. Then it can be usedin each helper. This is for example the case of a functor without arguments:
This code is inlined, so it will not cost anything at run-time.
Conclusion
This is it for this blog post. There is still a lot to talk about(I have not even mentioned QueuedConnection or thread safety yet), but I hope you found thisinterresting and that you learned here something that might help you as a programmer.
Update:The part 3 is available.
Home · All Classes · Modules |
The QObject class is the base class of all Qt objects. More...
Inherited by AbstractAudioOutput, Notifier, Effect, MediaController, MediaObject, QAbstractAnimation, QAbstractEventDispatcher, QAbstractItemDelegate, QAbstractItemModel, QAbstractMessageHandler, QAbstractNetworkCache, QAbstractState, QAbstractTextDocumentLayout, QAbstractTransition, QAbstractUriResolver, QAbstractVideoSurface, QAction, QActionGroup, QAssistantClient, QAudioInput, QAudioOutput, QButtonGroup, QClipboard, QCompleter, QCoreApplication, QDataWidgetMapper, QDBusAbstractAdaptor, QDBusAbstractInterface, QDBusPendingCallWatcher, QDBusServiceWatcher, QDeclarativeComponent, QDeclarativeContext, QDeclarativeEngine, QDeclarativeExpression, QDeclarativeExtensionPlugin, QDeclarativePropertyMap, QDesignerFormEditorInterface, QDesignerFormWindowManagerInterface, QDrag, QEventLoop, QExtensionFactory, QExtensionManager, QFileSystemWatcher, QFtp, QGesture, QGLShader, QGLShaderProgram, QGraphicsAnchor, QGraphicsEffect, QGraphicsItemAnimation, QGraphicsObject, QGraphicsScene, QGraphicsTransform, QHelpEngineCore, QHelpSearchEngine, QHttp, QHttpMultiPart, QInputContext, QIODevice, QItemSelectionModel, QLayout, QLibrary, QLocalServer, QMimeData, QMovie, QNetworkAccessManager, QNetworkConfigurationManager, QNetworkCookieJar, QNetworkSession, QObjectCleanupHandler, QPluginLoader, QPyDeclarativePropertyValueSource, QPyDesignerContainerExtension, QPyDesignerCustomWidgetCollectionPlugin, QPyDesignerCustomWidgetPlugin, QPyDesignerMemberSheetExtension, QPyDesignerPropertySheetExtension, QPyDesignerTaskMenuExtension, QPyTextObject, QScriptEngine, QScriptEngineDebugger, QSessionManager, QSettings, QSharedMemory, QShortcut, QSignalMapper, QSocketNotifier, QSound, QSqlDriver, QStyle, QSvgRenderer, QSyntaxHighlighter, QSystemTrayIcon, QTcpServer, QTextDocument, QTextObject, QThread, QThreadPool, QTimeLine, QTimer, QTranslator, QUndoGroup, QUndoStack, QValidator, QWebFrame, QWebHistoryInterface, QWebPage, QWebPluginFactory and QWidget.
Methods
- bool blockSignals (self, bool b)
- list-of-QObject children (self)
- bool connect (self, QObject, SIGNAL(), SLOT(), Qt.ConnectionType = Qt.AutoConnection)
- customEvent (self, QEvent)
- disconnectNotify (self, SIGNAL() signal)
- dumpObjectTree (self)
- emit (self, SIGNAL(), ...)
- bool eventFilter (self, QObject, QEvent)
- QObject findChild (self, type type, QString name = QString())
- QObject findChild (self, tuple types, QString name = QString())
- list-of-QObject findChildren (self, type type, QString name = QString())
- list-of-QObject findChildren (self, tuple types, QString name = QString())
- list-of-QObject findChildren (self, type type, QRegExp regExp)
- list-of-QObject findChildren (self, tuple types, QRegExp regExp)
- installEventFilter (self, QObject)
- killTimer (self, int id)
- moveToThread (self, QThread thread)
- QObject parent (self)
- pyqtConfigure (self, object)
- removeEventFilter (self, QObject)
- int senderSignalIndex (self)
- setParent (self, QObject)
- bool signalsBlocked (self)
- QThread thread (self)
- QString tr (self, str sourceText, str disambiguation = None, int n = -1)
- QString trUtf8 (self, str sourceText, str disambiguation = None, int n = -1)
Static Methods
- bool connect (QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType = Qt.AutoConnection)
- bool connect (QObject, SIGNAL(), callable, Qt.ConnectionType = Qt.AutoConnection)
- bool disconnect (QObject, SIGNAL(), QObject, SLOT())
Special Methods
- object __getattr__ (self, str name)
Qt Signals
Static Members
- QMetaObject staticMetaObject
Detailed Description
The QObject class is the base class of all Qt objects.
QObject is the heart of the Qt ObjectModel. The central feature in this model is a very powerfulmechanism for seamless object communication called signals and slots. Youcan connect a signal to a slot with connect() and destroy the connectionwith disconnect(). To avoidnever ending notification loops you can temporarily block signalswith blockSignals(). Theprotected functions connectNotify() and disconnectNotify() make itpossible to track connections.
QObjects organizethemselves in object trees. When youcreate a QObject with another object as parent, the object willautomatically add itself to the parent's children() list. The parent takesownership of the object; i.e., it will automatically delete itschildren in its destructor. You can look for an object by name andoptionally type using findChild() or findChildren().
Every object has an objectName() and its class namecan be found via the corresponding metaObject() (see QMetaObject.className()). You candetermine whether the object's class inherits another class in theQObject inheritance hierarchy by using the inherits() function.
When an object is deleted, it emits a destroyed() signal. You can catch thissignal to avoid dangling references to QObjects.
QObjects can receiveevents through event() and filterthe events of other objects. See installEventFilter() andeventFilter() for details. Aconvenience handler, childEvent(), can be reimplemented tocatch child events.
Last but not least, QObject provides the basic timer support inQt; see QTimer for high-level support fortimers.
Notice that the Q_OBJECTmacro is mandatory for any object that implements signals, slots orproperties. You also need to run the MetaObject Compiler on the source file. We strongly recommend theuse of this macro in all subclasses of QObject regardless ofwhether or not they actually use signals, slots and properties,since failure to do so may lead certain functions to exhibitstrange behavior.
All Qt widgets inherit QObject. The convenience functionisWidgetType() returnswhether an object is actually a widget. It is much faster thanqobject_cast<QWidget *>(obj) orobj->inherits('QWidget').
Some QObject functions, e.g. children(), return a QObjectList. QObjectList is a typedef forQList<QObject *>.
Thread Affinity
A QObject instance is said to have a thread affinity, orthat it lives in a certain thread. When a QObject receives aqueued signal or aposted event,the slot or event handler will run in the thread that the objectlives in.
Note: If a QObject has no thread affinity (that is, ifthread() returns zero), or if itlives in a thread that has no running event loop, then it cannotreceive queued signals or posted events.
By default, a QObject lives in the thread in which it iscreated. An object's thread affinity can be queried using thread() and changed using moveToThread().
All QObjects mustlive in the same thread as their parent. Consequently:
li
setParent() will fail if the twoQObjects involved livein different threads. li
Whena QObject is moved to another thread, all its children will beautomatically moved too. li
moveToThread() will fail ifthe QObject has a parent. li
If QObjects are created within QThread.run(), they cannot become childrenof the QThread object because theQThread does not live in the thread thatcalls QThread.run().
Note: A QObject's member variables do notautomatically become its children. The parent-child relationshipmust be set by either passing a pointer to the child's constructor, or by calling setParent(). Without this step, theobject's member variables will remain in the old thread whenmoveToThread() iscalled.
No copy constructor or assignment operator
QObject has neither a copy constructor nor an assignmentoperator. This is by design. Actually, they are declared, but in aprivate section with the macro Q_DISABLE_COPY(). In fact, all Qtclasses derived from QObject (direct or indirect) use this macro todeclare their copy constructor and assignment operator to beprivate. The reasoning is found in the discussion on Identity vs Value on the QtObject Model page.
The main consequence is that you should use pointers to QObject(or to your QObject subclass) where you might otherwise be temptedto use your QObject subclass as a value. For example, without acopy constructor, you can't use a subclass of QObject as the valueto be stored in one of the container classes. You must storepointers.
Auto-Connection
Qt's meta-object system provides a mechanism to automaticallyconnect signals and slots between QObject subclasses and theirchildren. As long as objects are defined with suitable objectnames, and slots follow a simple naming convention, this connectioncan be performed at run-time by the QMetaObject.connectSlotsByName()function.
uic generates code that invokes thisfunction to enable auto-connection to be performed between widgetson forms created with Qt Designer. More information aboutusing auto-connection with Qt Designer is given in theUsing a Designer UI File inYour Application section of the Qt Designer manual.
Dynamic Properties
From Qt 4.2, dynamic properties can be added to and removed fromQObject instances at run-time. Dynamic properties do not need to bedeclared at compile-time, yet they provide the same advantages asstatic properties and are manipulated using the same API - usingproperty() to read them andsetProperty() to writethem.
From Qt 4.3, dynamic properties are supported by Qt Designer,and both standard Qt widgets and user-created forms can be givendynamic properties.
Internationalization (i18n)
All QObject subclasses support Qt's translation features, makingit possible to translate an application's user interface intodifferent languages.
To make user-visible text translatable, it must be wrapped incalls to the tr() function. This isexplained in detail in the Writing Source Code forTranslation document.
Method Documentation
QObject.__init__ (self, QObjectparent = None)
The parent argument, if not None, causes self to be owned by Qt instead of PyQt.
Constructs an object with parent object parent.
The parent of an object may be viewed as the object's owner. Forinstance, a dialog box is the parent ofthe OK and Cancel buttons it contains.
The destructor of a parent object destroys all childobjects.
Setting parent to 0 constructs an object with no parent.If the object is a widget, it will become a top-level window.
See alsoparent(),findChild(), and findChildren().
bool QObject.blockSignals (self, bool b)
The return value is the previous value of signalsBlocked().
Note that the destroyed()signal will be emitted even if the signals for this object havebeen blocked.
See alsosignalsBlocked().
QObject.childEvent (self, QChildEvent)
This event handler can be reimplemented in a subclass to receivechild events. The event is passed in the eventparameter.
QEvent.ChildAdded andQEvent.ChildRemoved events aresent to objects when children are added or removed. In both casesyou can only rely on the child being a QObject, or if isWidgetType() returns true, aQWidget. (This is because, in theChildAdded case, the child isnot yet fully constructed, and in the ChildRemoved case it might have beendestructed already).
QEvent.ChildPolished eventsare sent to widgets when children are polished, or when polishedchildren are added. If you receive a child polished event, thechild's construction is usually completed. However, this is notguaranteed, and multiple polish events may be delivered during theexecution of a widget's constructor.
For every child widget, you receive one ChildAdded event, zero or more ChildPolished events, and one ChildRemoved event.
The ChildPolished event isomitted if a child is removed immediately after it is added. If achild is polished several times during construction anddestruction, you may receive several child polished events for thesame child, each time with a different virtual table.
See alsoevent().
list-of-QObject QObject.children (self)
Returns a list of child objects. The QObjectList class is definedin the <QObject> header file as the following:
The first child added is the first object in the list and the last childadded is the last object in the list,i.e. new children are appended at the end.
Note that the list order changes when QWidget children are raised or lowered. A widget that is raised becomesthe last object in the list, and a widget that is lowered becomesthe first object in the list.
See alsofindChild(), findChildren(), parent(), and setParent().
bool QObject.connect (QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType = Qt.AutoConnection)
Creates a connection of the given type from thesignal in the sender object to the method inthe receiver object. Returns true if the connectionsucceeds; otherwise returns false.
You must use the SIGNAL() and SLOT() macroswhen specifying the signal and the method, forexample:
This example ensures that the label always displays the currentscroll bar value. Note that the signal and slots parameters mustnot contain any variable names, only the type. E.g. the followingwould not work and return false:
A signal can also be connected to another signal:
In this example, the MyWidget constructor relays asignal from a private member variable, and makes it available undera name that relates to MyWidget.
A signal can be connected to many slots and signals. Manysignals can be connected to one slot.
If a signal is connected to several slots, the slots areactivated in the same order as the order the connection was made,when the signal is emitted.
The function returns true if it successfully connects the signalto the slot. It will return false if it cannot create theconnection, for example, if QObject isunable to verify the existence of either signal ormethod, or if their signatures aren't compatible.
By default, a signal is emitted for every connection you make;two signals are emitted for duplicate connections. You can breakall of these connections with a single disconnect() call. If you pass theQt.UniqueConnectiontype, the connection will only be made if it is not aduplicate. If there is already a duplicate (exact same signal tothe exact same slot on the same objects), the connection will failand connect will return false.
The optional type parameter describes the type ofconnection to establish. In particular, it determines whether aparticular signal is delivered to a slot immediately or queued fordelivery at a later time. If the signal is queued, the parametersmust be of types that are known to Qt's meta-object system, becauseQt needs to copy the arguments to store them in an event behind thescenes. If you try to use a queued connection and get the errormessage
call qRegisterMetaType() toregister the data type before you establish the connection.
Note: This function is thread-safe.
See alsodisconnect(), sender(), qRegisterMetaType(), andQ_DECLARE_METATYPE().
bool QObject.connect (QObject, SIGNAL(), callable, Qt.ConnectionType = Qt.AutoConnection)
Creates a connection of the given type from thesignal in the sender object to the method inthe receiver object. Returns true if the connectionsucceeds; otherwise returns false.
This function works in the same way as connect(const QObject *sender, const char *signal, constQObject *receiver, const char *method,Qt.ConnectionType type)but it uses QMetaMethod to specifysignal and method.
This function was introduced in Qt 4.8.
See also connect(const QObject *sender, const char*signal, const QObject *receiver, const char *method,Qt.ConnectionType type).
Qt No Such Slot In Derived Classroom
bool QObject.connect (self, QObject, SIGNAL(), SLOT(), Qt.ConnectionType = Qt.AutoConnection)
This function overloads connect().
Connects signal from the sender object to thisobject's method.
Equivalent to connect(sender, signal,this, method, type).
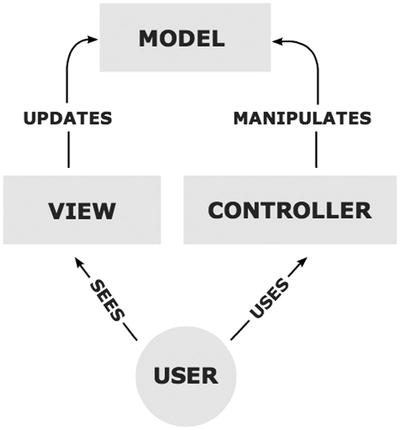
Every connection you make emits a signal, so duplicateconnections emit two signals. You can break a connection usingdisconnect().
Note: This function is thread-safe.
See alsodisconnect().
QObject.connectNotify (self, SIGNAL() signal)
If you want to compare signal with a specific signal, useQLatin1String and theSIGNAL() macro as follows:
If the signal contains multiple parameters or parameters thatcontain spaces, call QMetaObject.normalizedSignature()on the result of the SIGNAL() macro.
Warning: This function violates the object-orientedprinciple of modularity. However, it might be useful when you needto perform expensive initialization only if something is connectedto a signal.
See alsoconnect() anddisconnectNotify().
QObject.customEvent (self, QEvent)
This event handler can be reimplemented in a subclass to receivecustom events. Custom events are user-defined events with a typevalue at least as large as the QEvent.User item of the QEvent.Type enum, and is typically aQEvent subclass. The event is passed inthe event parameter.
See alsoevent() andQEvent.
QObject.deleteLater (self)
The object will be deleted when control returns to the eventloop. If the event loop is not running when this function is called(e.g. deleteLater() is called on an object before QCoreApplication.exec()), theobject will be deleted once the event loop is started. IfdeleteLater() is called after the main event loop has stopped, theobject will not be deleted. Since Qt 4.8, if deleteLater() iscalled on an object that lives in a thread with no running eventloop, the object will be destroyed when the thread finishes.
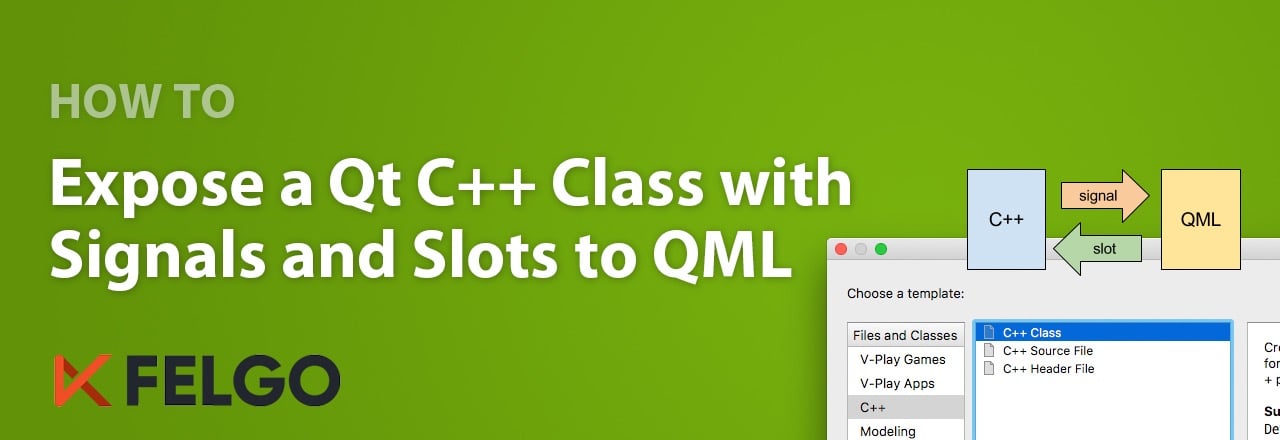
Note that entering and leaving a new event loop (e.g., byopening a modal dialog) will not perform the deferreddeletion; for the object to be deleted, the control must return tothe event loop from which deleteLater() was called.
Note: It is safe to call this function more than once;when the first deferred deletion event is delivered, any pendingevents for the object are removed from the event queue.
See alsodestroyed()and QPointer.
bool QObject.disconnect (QObject, SIGNAL(), QObject, SLOT())
Disconnects signal in object sender frommethod in object receiver. Returns true if theconnection is successfully broken; otherwise returns false.
A signal-slot connection is removed when either of the objectsinvolved are destroyed.
disconnect() is typically used in three ways, as the followingexamples demonstrate.
- Disconnect everything connected to an object's signals:
equivalent to the non-static overloaded function
- Disconnect everything connected to a specific signal:
equivalent to the non-static overloaded function
- Disconnect a specific receiver:
equivalent to the non-static overloaded function
0 may be used as a wildcard, meaning 'any signal', 'anyreceiving object', or 'any slot in the receiving object',respectively.
The sender may never be 0. (You cannot disconnect signalsfrom more than one object in a single call.)
If signal is 0, it disconnects receiver andmethod from any signal. If not, only the specified signal isdisconnected.
If receiver is 0, it disconnects anything connected tosignal. If not, slots in objects other than receiverare not disconnected.
If method is 0, it disconnects anything that is connectedto receiver. If not, only slots named method will bedisconnected, and all other slots are left alone. The methodmust be 0 if receiver is left out, so you cannot disconnecta specifically-named slot on all objects.
Note: This function is thread-safe.
See alsoconnect().
bool QObject.disconnect (QObject, SIGNAL(), callable)
Disconnects signal in object sender frommethod in object receiver. Returns true if theconnection is successfully broken; otherwise returns false.
This function provides the same possibilities likedisconnect(const QObject *sender, constchar *signal, const QObject *receiver,const char *method) but uses QMetaMethod to represent the signal and themethod to be disconnected.
Additionally this function returnsfalse and no signals and slotsdisconnected if:
- signal is not a member of sender class or one of itsparent classes.
- method is not a member of receiver class or one of itsparent classes.
- signal instance represents not a signal.
QMetaMethod() may be used as wildcard in the meaning 'anysignal' or 'any slot in receiving object'. In the same way 0 can beused for receiver in the meaning 'any receiving object'. Inthis case method should also be QMetaMethod(). senderparameter should be never 0.
This function was introduced in Qt 4.8.
See also disconnect(const QObject *sender, const char*signal, const QObject *receiver, const char *method).
QObject.disconnectNotify (self, SIGNAL() signal)
See connectNotify() foran example of how to compare signal with a specificsignal.
Warning: This function violates the object-orientedprinciple of modularity. However, it might be useful for optimizingaccess to expensive resources.
See alsodisconnect() and connectNotify().
QObject.dumpObjectInfo (self)
See alsodumpObjectTree().
QObject.dumpObjectTree (self)
See alsodumpObjectInfo().
list-of-QByteArray QObject.dynamicPropertyNames (self)
Returns the names of all properties that were dynamically addedto the object using setProperty().
This function was introduced in Qt 4.2.
QObject.emit (self, SIGNAL(), ...)
bool QObject.event (self, QEvent)
This virtual function receives events to an object and shouldreturn true if the event e was recognized and processed.
The event() function can be reimplemented to customize thebehavior of an object.
See alsoinstallEventFilter(),timerEvent(), QApplication.sendEvent(),QApplication.postEvent(),and QWidget.event().
bool QObject.eventFilter (self, QObject, QEvent)
Filters events if this object has been installed as an eventfilter for the watched object.
In your reimplementation of this function, if you want to filterthe event out, i.e. stop it being handled further, returntrue; otherwise return false.
Example:
Notice in the example above that unhandled events are passed tothe base class's eventFilter() function, since the base class mighthave reimplemented eventFilter() for its own internal purposes.
Warning: If you delete the receiver object in thisfunction, be sure to return true. Otherwise, Qt will forward theevent to the deleted object and the program might crash.
See alsoinstallEventFilter().
QObject QObject.findChild (self, type type, QString name = QString())
If there is more than one child matching the search, the mostdirect ancestor is returned. If there are several direct ancestors,it is undefined which one will be returned. In that case, findChildren() should be used.
This example returns a child QPushButton of parentWidget named'button1':
This example returns a QListWidget child ofparentWidget:
See alsofindChildren().
QObject QObject.findChild (self, tuple types, QString name = QString())
Qt No Such Slot In Derived Classes
list-of-QObject QObject.findChildren (self, type type, QString name = QString())
The following example shows how to find a list of child QWidgets of the specified parentWidgetnamed widgetname:
This example returns all QPushButtons that are childrenof parentWidget:
See alsofindChild().
list-of-QObject QObject.findChildren (self, tuple types, QString name = QString())
This function overloads findChildren().
Returns the children of this object that can be cast to type Tand that have names matching the regular expression regExp,or an empty list if there are no such objects. The search isperformed recursively.
list-of-QObject QObject.findChildren (self, type type, QRegExpregExp)
list-of-QObject QObject.findChildren (self, tuple types, QRegExpregExp)
bool QObject.inherits (self, str classname)
Returns true if this object is an instance of a class thatinherits className or a QObjectsubclass that inherits className; otherwise returnsfalse.
A class is considered to inherit itself.
Example:
If you need to determine whether an object is an instance of aparticular class for the purpose of casting it, consider usingqobject_cast<Type *>(object) instead.
See alsometaObject() and qobject_cast().
QObject.installEventFilter (self, QObject)
Installs an event filter filterObj on this object. Forexample:
An event filter is an object that receives all events that aresent to this object. The filter can either stop the event orforward it to this object. The event filter filterObjreceives events via its eventFilter() function. The eventFilter() function must returntrue if the event should be filtered, (i.e. stopped); otherwise itmust return false.
If multiple event filters are installed on a single object, thefilter that was installed last is activated first.
Qt No Such Slot In Derived Class C
Here's a KeyPressEater class that eats the key pressesof its monitored objects:
And here's how to install it on two widgets:
The QShortcut class, for example,uses this technique to intercept shortcut key presses.
Warning: If you delete the receiver object in youreventFilter() function, besure to return true. If you return false, Qt sends the event to thedeleted object and the program will crash.
Note that the filtering object must be in the same thread asthis object. If filterObj is in a different thread, thisfunction does nothing. If either filterObj or this objectare moved to a different thread after calling this function, theevent filter will not be called until both objects have the samethread affinity again (it is not removed).
See alsoremoveEventFilter(), eventFilter(), and event().
bool QObject.isWidgetType (self)
Calling this function is equivalent to callinginherits('QWidget'), except that it ismuch faster.
QObject.killTimer (self, int id)
The timer identifier is returned by startTimer() when a timer event isstarted.
See alsotimerEvent() and startTimer().
QMetaObject QObject.metaObject (self)
Returns a pointer to the meta-object of this object.
A meta-object contains information about a class that inheritsQObject, e.g. class name, superclassname, properties, signals and slots. Every QObject subclass that contains the Q_OBJECT macro will have ameta-object.
The meta-object information is required by the signal/slotconnection mechanism and the property system. The inherits() function also makes use ofthe meta-object.
If you have no pointer to an actual object instance but stillwant to access the meta-object of a class, you can use staticMetaObject.
Example:
See alsostaticMetaObject.
QObject.moveToThread (self, QThreadthread)
Changes the thread affinity for this object and its children.The object cannot be moved if it has a parent. Event processingwill continue in the targetThread.
To move an object to the main thread, use QApplication.instance() toretrieve a pointer to the current application, and then useQApplication.thread() toretrieve the thread in which the application lives. Forexample:
If targetThread is zero, all event processing for thisobject and its children stops.
Note that all active timers for the object will be reset. Thetimers are first stopped in the current thread and restarted (withthe same interval) in the targetThread. As a result,constantly moving an object between threads can postpone timerevents indefinitely.
A QEvent.ThreadChange eventis sent to this object just before the thread affinity is changed.You can handle this event to perform any special processing. Notethat any new events that are posted to this object will be handledin the targetThread.
Warning: This function is not thread-safe; thecurrent thread must be same as the current thread affinity. Inother words, this function can only 'push' an object from thecurrent thread to another thread, it cannot 'pull' an object fromany arbitrary thread to the current thread.
See alsothread().
QString QObject.objectName (self)
QObject QObject.parent (self)
Returns a pointer to the parent object.
See alsosetParent()and children().
QVariant QObject.property (self, str name)
Information about all available properties is provided throughthe metaObject() and dynamicPropertyNames().
See alsosetProperty(), QVariant.isValid(), metaObject(), and dynamicPropertyNames().
QObject.pyqtConfigure (self, object)
int QObject.receivers (self, SIGNAL() signal)
QObject.removeEventFilter (self, QObject)
Removes an event filter object obj from this object. Therequest is ignored if such an event filter has not beeninstalled.
All event filters for this object are automatically removed whenthis object is destroyed.
It is always safe to remove an event filter, even during eventfilter activation (i.e. from the eventFilter() function).
See alsoinstallEventFilter(),eventFilter(), and event().
QObject QObject.sender (self)
Returns a pointer to the object that sent the signal, if calledin a slot activated by a signal; otherwise it returns 0. Thepointer is valid only during the execution of the slot that callsthis function from this object's thread context.
The pointer returned by this function becomes invalid if thesender is destroyed, or if the slot is disconnected from thesender's signal.
Warning: This function violates the object-orientedprinciple of modularity. However, getting access to the sendermight be useful when many signals are connected to a singleslot.
Warning: As mentioned above, the return value of thisfunction is not valid when the slot is called via a Qt.DirectConnection from athread different from this object's thread. Do not use thisfunction in this type of scenario.
See alsosenderSignalIndex() andQSignalMapper.
int QObject.senderSignalIndex (self)
Returns the meta-method index of the signal that called thecurrently executing slot, which is a member of the class returnedby sender(). If called outside ofa slot activated by a signal, -1 is returned.
For signals with default parameters, this function will alwaysreturn the index with all parameters, regardless of which was usedwith connect(). For example, thesignal destroyed(QObject *obj = 0) will have two differentindexes (with and without the parameter), but this function willalways return the index with a parameter. This does not apply whenoverloading signals with different parameters.
Warning: This function violates the object-orientedprinciple of modularity. However, getting access to the signalindex might be useful when many signals are connected to a singleslot.
Warning: The return value of this function is not validwhen the slot is called via a Qt.DirectConnection from athread different from this object's thread. Do not use thisfunction in this type of scenario.
This function was introduced in Qt 4.8.
See alsosender(),QMetaObject.indexOfSignal(),and QMetaObject.method().
QObject.setObjectName (self, QString name)
QObject.setParent (self, QObject)
The QObject argument, if not None, causes self to be owned by Qt instead of PyQt.
Makes the object a child of parent.
See alsoparent() andQWidget.setParent().
bool QObject.setProperty (self, str name, QVariant value)
Information about all available properties is provided throughthe metaObject() and dynamicPropertyNames().
Dynamic properties can be queried again using property() and can be removed bysetting the property value to an invalid QVariant. Changing the value of a dynamicproperty causes a QDynamicPropertyChangeEventto be sent to the object.
Note: Dynamic properties starting with '_q_' are reservedfor internal purposes.
See alsoproperty(),metaObject(), and dynamicPropertyNames().
bool QObject.signalsBlocked (self)
See alsoblockSignals().
int QObject.startTimer (self, int interval)
A timer event will occur every interval millisecondsuntil killTimer() is called.If interval is 0, then the timer event occurs once everytime there are no more window system events to process.
The virtual timerEvent()function is called with the QTimerEvent event parameter class when atimer event occurs. Reimplement this function to get timerevents.
If multiple timers are running, the QTimerEvent.timerId() can be usedto find out which timer was activated.
Example:
Note that QTimer's accuracy depends onthe underlying operating system and hardware. Most platformssupport an accuracy of 20 milliseconds; some provide more. If Qt isunable to deliver the requested number of timer events, it willsilently discard some.
The QTimer class provides a high-levelprogramming interface with single-shot timers and timer signalsinstead of events. There is also a QBasicTimer class that is more lightweightthan QTimer and less clumsy than usingtimer IDs directly.
See alsotimerEvent(), killTimer(), and QTimer.singleShot().
QThread QObject.thread (self)
Returns the thread in which the object lives.
See alsomoveToThread().
QObject.timerEvent (self, QTimerEvent)
Qt No Such Slot In Derived Class Example
This event handler can be reimplemented in a subclass to receivetimer events for the object.
QTimer provides a higher-levelinterface to the timer functionality, and also more generalinformation about timers. The timer event is passed in theevent parameter.
See alsostartTimer(), killTimer(), and event().
QString QObject.tr (self, str sourceText, str disambiguation = None, int n = -1)
See Writing Source Codefor Translation for a detailed description of Qt's translationmechanisms in general, and the Disambiguationsection for information on disambiguation.
Warning: This method is reentrant only if all translatorsare installed before calling this method. Installing orremoving translators while performing translations is notsupported. Doing so will probably result in crashes or otherundesirable behavior.
See alsotrUtf8(),QApplication.translate(),QTextCodec.setCodecForTr(),and Internationalization withQt.
QString QObject.trUtf8 (self, str sourceText, str disambiguation = None, int n = -1)
See alsotr(), QApplication.translate(),and Internationalization withQt.
object QObject.__getattr__ (self, str name)
Qt Signal Documentation
void destroyed (QObject* = 0)
See alsodeleteLater() and QPointer.
Member Documentation
QMetaObject staticMetaObject
This member should be treated as a constant.
This variable stores the meta-object for the class.
A meta-object contains information about a class that inheritsQObject, e.g. class name, superclassname, properties, signals and slots. Every class that contains theQ_OBJECT macro will also have ameta-object.
The meta-object information is required by the signal/slotconnection mechanism and the property system. The inherits() function also makes use ofthe meta-object.
If you have a pointer to an object, you can use metaObject() to retrieve themeta-object associated with that object.
Example:
See alsometaObject().
PyQt 4.11.4 for X11 | Copyright © Riverbank Computing Ltd and The Qt Company 2015 | Qt 4.8.7 |